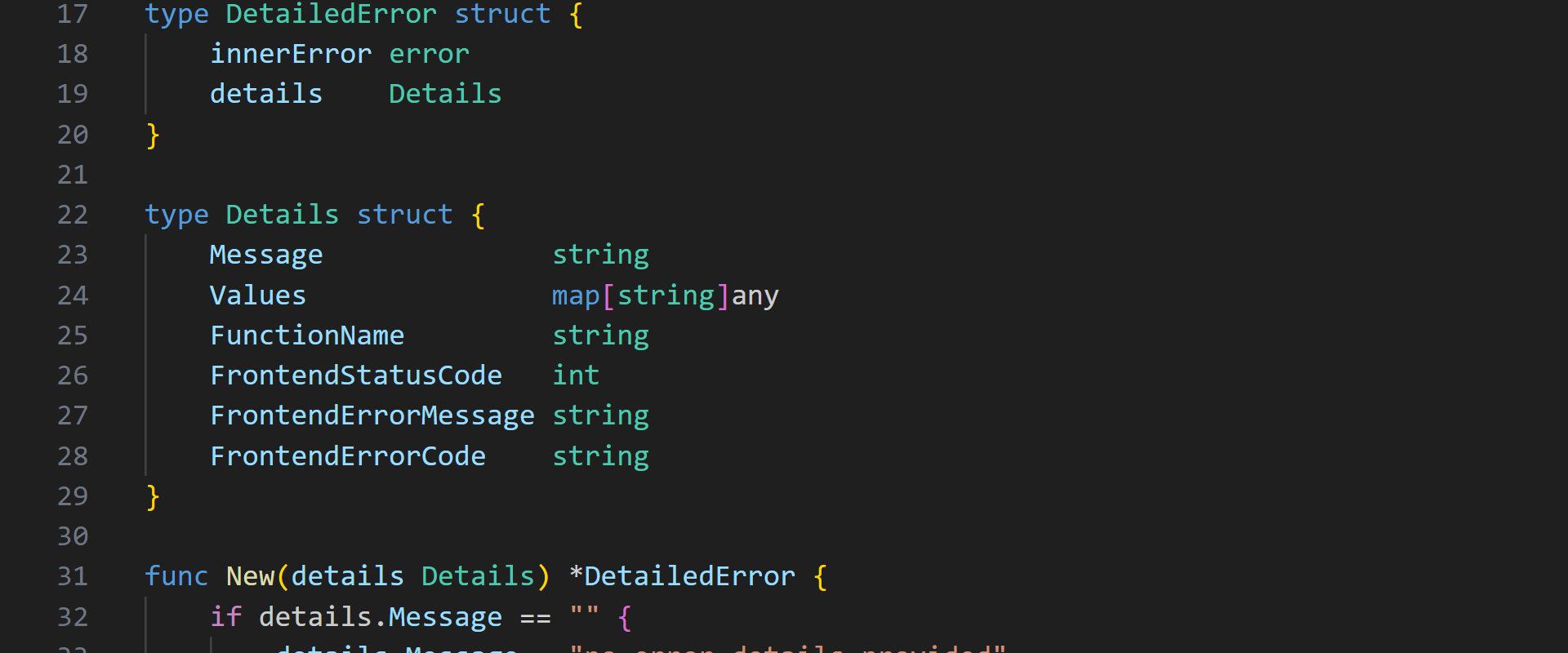
Web error handling concept
Published: Dec 22, 2024
Table of content
- Defining the goals of the concept
- Defining how errors are transmitted
- Handling errors in the backend
- Transferring error to the frontend
- Handling errors in the frontend
- Conclusion
• • •
Transferring error to the frontend
The next step would be transferring the error to the frontend.
For this, you need to walk down the DetailedError chain and search for frontend information.
Here is an example of how it could look like:
// Example in go
func sendErrorResponse(w http.ResponseWriter, err error) {
errorCode := "ERROR_INTERNAL_SERVER_ERROR"
errorMessage := "Internal server error"
statusCode := http.StatusInternalServerError
for {
detailedError, ok := err.(&DetailedError)
if !ok {
break
}
hasFrontendInfo := false
if detailedError.FrontendErrorCode {
errorCode = detailedError.FrontendErrorCode
hasFrontendInfo = true
}
if detailedError.FrontendErrorMessage {
errorMessage = detailedError.FrontendErrorMessage
hasFrontendInfo = true
}
if detailedError.FrontendStatusCode {
statusCode = detailedError.FrontendStatusCode
hasFrontendInfo = true
}
if hasFrontendInfo {
// break here, because we want the most recent info
break
}
e = detailedError.InnerError
}
w.Header().Set("Content-Type", "application/json")
w.WriteHeader(statusCode)
fmt.Fprintf(w, toJsonString(map[string]any{
"errorCode": errorCode,
"error": errorMessage,
}))
}
Or when you prefer TS:
// Example in TS
function sendErrorResponse(res: Response, e: unknown) {
let errorCode = "ERROR_INTERNAL_SERVER_ERROR"
let errorMessage = "Internal server error"
let statusCode = 500
let curentError: unknown = e
while (e instanceof DetailedError) {
let hasFrontendInfo = false
if (detailedError.frontendErrorCode) {
errorCode = detailedError.frontendErrorCode
hasFrontendInfo = true
}
if (detailedError.frontendErrorMessage) {
errorMessage = detailedError.frontendErrorMessage
hasFrontendInfo = true
}
if (detailedError.frontendStatusCode) {
statusCode = detailedError.frontendStatusCode
hasFrontendInfo = true
}
if (hasFrontendInfo) {
// break here, because we want the most recent info
break
}
e = e.innerError
}
res.status(statusCode).json({
"errorCode": errorCode,
"error": errorMessage,
});
}